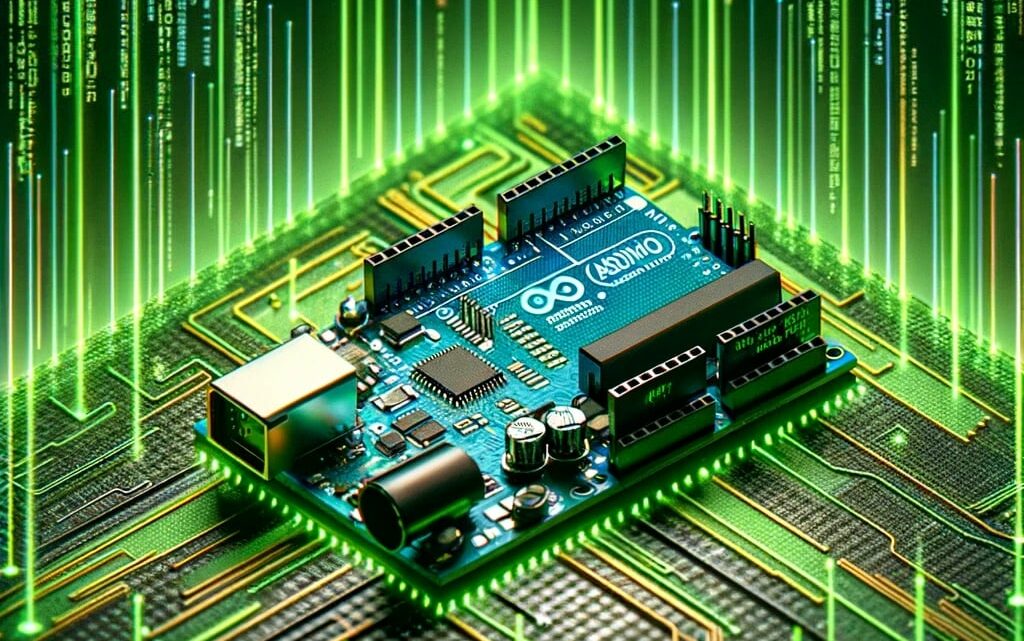
Arduino Assembler Tutorial : Our First Programm
Wednesday January 17th, 2024In this series I want to show you, how to use the Assembler (ASM) language on your Arduino and AVR. Instead of using plain assembly however, I will show you how to merge it with your C++ Code. Keep in mind, that ASM code is not very portable. This tutorial is only for AVR processors (like the Atmega168 or Atmega328 for example). Your code will not work on an Arduino Due (except for trivial cases), since it uses an ARM processor with vastly different architecture. It is also assumed, that you use the AVR-GCC compiler, which is the standard compiler used by the Arduino IDE. Anyway, a good place to start is to modify the “Blink” example sketch which comes with the Arduino.
First of all, you should know that there are (at least) 2 ways to merge C/C++ Code with ASM. The first one ist to use Inline Assembler and the second one is to write function calls in assembly. I will show you the second option, since Inline Assembler uses (in my opinion) a horrible syntax which makes the already hard to read ASM even harder to debug.
The workflow is similar to writing a library. We will need to create a header file in which we will declare our functions and a second .S file which will contain our implementation. You can find all files on Github: https://gist.github.com/madgyver/92540e0f8621bc8b0566
Lets get started:
- Create a new folder called “Assembler” in your library folder
- In this folder, create the files “ASM_Blink.H” and “ASM_Blink.S”
ASM_Blink.H contains our declarations and looks like this:
void ASM_digitalWrite(char pin); void ASM_delay();
This part:
extern "C" { }
is a small detail, that makes AVR-GCC compile the declarations as C Code instead of C++. It smoothes out some issues with the function calls that I don’t understand completely but has never been a problem so far that Yamitenshi at reddit explained to me as follows:
Because C++ allows overloading (defining multiple functions with the same name but different arguments), function names are mangled, meaning (as a simple example)
int foo(int a, int b)
might end up being namedfoo_riaiai
when the compiler is done with it, as opposed toint foo(int a)
might be calledfoo_riai
so the two can be told apart.C does not allow this – therefore both
int foo(int a, int b)
andvoid foo(int a, char *b, float c)
will be namedfoo
when the compiler is done with it.
extern "C" {}
makes sure that the compiler knows that whatever library has defined foo was compiled in such a way that it’s still calledfoo
, and not some variation thereof, which has the added benefit of allowing you to definefoo
in assembly, because you know the linker will be looking forfoo
, and notfoo_riaiai
.
Besides that, this is a pretty standard declaration of 2 functions.
The file ASM_Blink.S contains the implementation:
.global ASM_digitalWrite ASM_digitalWrite: out 0x05,r24 ;write pin_value to port reti ; delay for 1 sec .global ASM_delay ASM_delay: ldi R17, 0x53 delay_loop: ldi R18, 0xFB delay_loop1: ldi R19, 0xFF delay_loop2: dec R19 brne delay_loop2 dec R18 brne delay_loop1 dec R17 brne delay_loop ret
The directive
.global
makes the following function names globally visible so that the linker can find it. The function ASM_digitalWrite uses a 1 Byte argument, which will be placed into register R24 by AVR-GCC. The function itself will then output that byte to PortB. You may be wondering, why AVR-GCC places our argument at R24 of all places. Turns out, there is an algorithm it uses to determine the placement of arguments, which you can read up on in the official wiki..
It is a little bit complicated, when you read it for the first time.
For our example it boils down to: Our function ASM_digitalWrite only uses one argument with the size of 1 Byte. Since 1 is an odd number, we round it to 2 and subtract that from R26, which gives us R24 where our argument will reside.
Now we just need to put everything inside a sketch to make it work on our Arduino:
#include "ASM_Blink.h" // change LEDPIN based on your schematic #define LEDPIN PINB1 void setup(){ pinMode(13, OUTPUT); } void loop(){ ASM_digitalWrite(0x20); ASM_delay(); ASM_digitalWrite(0x00); ASM_delay(); }
As you can see, it is not different from including and using a “normal” C++ library.